Design Patterns: Elements of Reusable Object-Oriented Software Book
Table Of Content
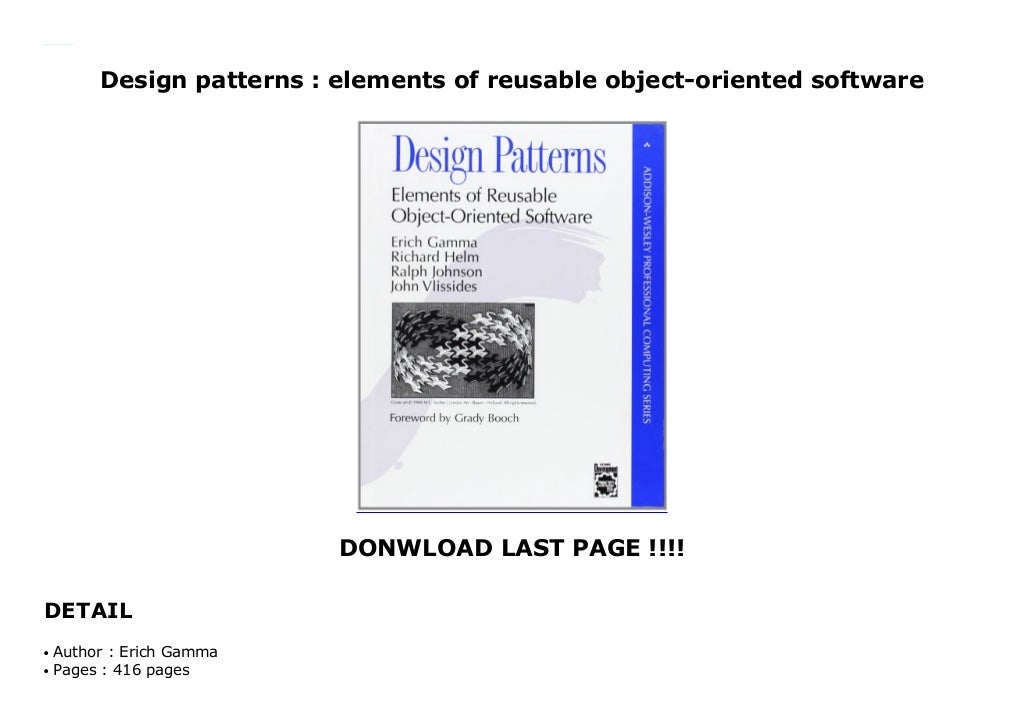
A singleton class ensures that only one instance of the class can be created. The pattern is often called an anti-pattern because it may lead to high coupling of components. The mediator pattern is used to reduce coupling between classes that communicate with each other. Instead of classes communicating directly, and thus requiring knowledge of their implementation, the classes send messages via a mediator object. The chain of responsibility pattern is a design pattern that defines a linked list of handlers, each of which is able to process requests.
Erich Gamma, Grady Booch, Richard Helm ...more
Sometimes acquaintance is called 'association' or the 'using' relationship. Acquaintance objects may request operations of each other, but they are not responsible for each other. Acquaintance is a weaker relationship than aggregation and suggests much looser coupling between objects, which can often be desirable for maximum maintainability in designs. They warn that the implementation of a subclass can become so bound up with the implementation of its parent class that any change in the parent's implementation will force the subclass to change. Furthermore, they claim that a way to avoid this is to inherit only from abstract classes—but then, they point out that there is minimal code reuse. Use of an interface also leads to dynamic binding and polymorphism, which are central features of object-oriented programming.
Gunnar Peipman – Programming Blog
The interpreter pattern is used to define the grammar for instructions that form part of a language or notation, whilst allowing the grammar to be easily extended. The flyweight pattern is used to reduce the memory and resource usage for complex models containing many hundreds, thousands or hundreds of thousands of similar objects. The prototype pattern is used to instantiate a new object by copying all of the properties of an existing object, creating an independent clone.
Check it out now on O’Reilly
Before a design is finished, they usually try to reuse it several times, modifying it each time. The design patterns require neither unusual language features nor amazing programming tricks with which to astound your friends and managers. All can be implemented in standard object-oriented languages, though they might take a little more work than ad hoc solutions. But the extra effort invariably pays dividends in increased flexibility and reusability. The authors begin by describing what patterns are and how they can help you design object-oriented software. With Design Patterns as your guide, you will learn how these important patterns fit into the software development process, and how you can leverage them to solve your own design problems most efficiently.
About O’Reilly
18 Best Software Engineering Books - Built In
18 Best Software Engineering Books.
Posted: Wed, 24 May 2023 16:01:25 GMT [source]
AB - Capturing a wealth of experience about the design of object-oriented software, four top-notch designers present a catalog of simple and succinct solutions to commonly occurring design problems. They then go on to systematically name, explain, evaluate, and catalog recurring designs in object-oriented systems. All patterns are compiled from real systems and are based on real-world examples. Each pattern also includes code that demonstrates how it may be implemented in object-oriented programming languages like C++ or Smalltalk.
Download the O’Reilly App
When a request is submitted to the chain, it is passed to the first handler in the list that is able to process it. The shape of a program should reflect only the problem it needs to solve. Any other regularity in the code is a sign, to me at least, that I'm using abstractions that aren't powerful enough-- often that I'm generating by hand the expansions of some macro that I need to write. The composite pattern describes a way to create tree structures using objects and object groups.
References
When this book was published first it was revolutionary because it contained still undocumented knowledge from software development field that needed some systematic work and organization on it. Today we see these patterns in many programs and developers on different platforms are more and more aware of these first defined design patterns. Designing object-oriented software is hard, and designing reusable object-oriented software is even harder. You must find pertinent objects, factor them into classes at the right granularity, define class interfaces and inheritance hierarchies, and establish key relationships among them. Your design should be specific to the problem at hand but also general enough to address future problems and requirements. Experienced object-oriented designers will tell you that a reusable and flexible design is difficult if not impossible to get “right” the first time.
Search code, repositories, users, issues, pull requests...
Patterns are more like receipts – they point you to right direction but they don’t guarantee that they are the solution you are looking for. Same way you use receipts in cooking book – you know what you want, book gives just points you to right direction and it is up to you to get there. The state pattern is used to alter the behaviour of an object as its internal state changes. The pattern allows the class for an object to apparently change at run-time.
Interface for Processes
A. Leftover Patterns - Head First Design Patterns, 2nd Edition [Book] - O'Reilly Media
A. Leftover Patterns - Head First Design Patterns, 2nd Edition .
Posted: Wed, 22 Sep 2021 21:19:37 GMT [source]
The advantage of this technique is that the requests can be queued, logged or implemented to support undo/redo. Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day. O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.
The clients can access and use individual objects and compositions in the same manner. The authors also discuss so-called parameterized types, which are also known as generics (Ada, Eiffel, Java, C#, VB.NET, and Delphi) or templates (C++). These allow any type to be defined without specifying all the other types it uses—the unspecified types are supplied as 'parameters' at the point of use.
An abstract factory offers the interface for creating a set of related or dependant objects without explicitly specifying their classes. An object with methods to create objects without specifying the exact class that will be created. Depending on the concrete factory implementation objects with different classes are created.
Hence they aren't the designs people They reflect untold redesign and recoding as developers have struggled for greater reuse and flexibility in their software.Design patterns capture these solutions in a succinct and easily applied form. This book assumes you are reasonably proficient in at least one object-oriented programming language, and you should have some experience in object-oriented design as well. You definitely shouldn't have to rush to the nearest dictionary the moment we mention 'types' and'polymorphism,' or 'interface' as opposed to 'implementation' inheritance. You definitely shouldn't have to rush to the nearest dictionary the moment we mention "types" and"polymorphism," or "interface" as opposed to "implementation" inheritance. The authors refer to inheritance as white-box reuse, with white-box referring to visibility, because the internals of parent classes are often visible to subclasses. Design Patterns is golden classics of software design books written by Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides (aka Gang of Four – GoF).
More light-hearted criticism has included a show trial at the 1999 OOPSLA meeting,[11] and a parody of the format by Jim Coplien entitled "Kansas City Air Conditioner". The book started at a birds-of-a-feather session at the 1990 OOPSLA meeting, "Towards an Architecture Handbook", where Erich Gamma and Richard Helm met and discovered their common interest. They were later joined by Ralph Johnson and John Vlissides.[6] The book was originally published on 21 October 1994, with a 1995 copyright, and was made available to the public at the 1994 OOPSLA meeting.
In their parlance, toolkits are the object-oriented equivalent of subroutine libraries, whereas a 'framework' is a set of cooperating classes that make up a reusable design for a specific class of software. They state that applications are hard to design, toolkits are harder, and frameworks are the hardest to design. The authors further distinguish between 'Aggregation', where one object 'has' or 'is part of' another object (implying that an aggregate object and its owner have identical lifetimes) and acquaintance, where one object merely 'knows of' another object.
Comments
Post a Comment